This is a guest post from Cypress Ambassador Kailash Pathak!
Kailash Pathak is a Sr. QA Lead and 3Pillar Global. Read his blogs at qaautomationlabs.com/blogs. Kailash is 2x AWS | PMI-ACP, ITIL, and PRINCE2 practitioner certified.
This post is the first of a series that explores API testing with Cypress. Stay tuned on our blog and Twitter for more!
What is API Automation?
The process of automatically testing and verifying the functionality of Application Programming Interfaces (APIs) is known as API Automation. This helps to ensure that the APIs are functioning as intended and reduces the time and effort required for manual testing.
API Automation is achieved by using software tools that send requests to the API, analyze the responses, and compare them to the expected results. The goal of API automation is to improve the reliability and consistency of the testing process, thus saving time and resources.
What Is a REST API?
REST API, which stands for Representational State Transfer API, which is a web-based architecture that follows web standards for building web services. It is a common way for communicating between clients and servers over the internet.
REST APIs use HTTP requests to POST (create), PUT (update), GET (read), and DELETE (delete) to manage data. API automation allows for efficient and thorough testing of REST APIs. This is achieved by making multiple API calls and verifying the responses, helping to identify and resolve bugs and issues early on in the development process.
A REST API Example
Suppose you have a website that lists information about books and you want to allow other applications to retrieve this information. You can build a REST API for this.
Here’s how it works:
Endpoint: A client application would make a request to a specific URL, known as an endpoint, that represents a collection of books. For example: “https://www.example.com/api/books".
HTTP Methods: The client would use one of the following HTTP methods for making a request:
- GET: To retrieve information about a book or a collection of books.
- POST: To add a new book to the collection.
- PUT: To update information about a book.
- DELETE: To remove a book from the collection.
Response: The server would respond with data in a specific format, such as JSON, that the client can use to retrieve the information about books. For example, a client could make a GET request to “https://www.example.com/api/books" to retrieve a list of all books in the collection. The server would respond with a JSON payload that includes information about each book, such as its title, author, and ISBN number.
This example shows how the REST API provides a standardized, programmatic way for client applications to interact with the website’s data about books.
Cypress For API Automation
Cypress is a JavaScript-based testing framework for web applications. It allows you to write end-to-end tests for your application’s UI and APIs. This makes it a great tool for API automation.
Here’s an example of how to use Cypress for API testing:
- Install Cypress:
npm install cypress --save-dev
- Create a test files for GET, POST, PUT and DELETE methods under
cypress/e2e/cypress_api_tc.cy.js
GET Method
Let's see how GET methods work here to fetch user details.
it("GET API testing Using Cypress API Plugin", () => {
cy.request("GET", "https://reqres.in/api/users?page=2").should((response) => {
expect(response.status).to.eq(200);
});
});
POST Method
Here's how POST methods work to add user details.
it("POST API testing Using Cypress API Plugin", () => {
cy.request("POST", "https://reqres.in/api/users", {
name: "morpheus",
job: "leader",
}).should((response) => {
expect(response.status).to.eq(201);
});
});
PUT Method
Let's see how PUT methods work to update user details.
it("PUT API testing Using Flip Plugin", () => {
cy.request("PUT", "https://reqres.in/api/users/2", {
name: "QAAutomationLabs",
job: "QA Automation Engg",
}).should((response) => {
expect(response.status).to.eq(200);
});
});
Delete Method
Let's see how DELETE methods work to delete user details.
it("DELETE API testing Using Cypress API Plugin", () => {
cy.request("DELETE", "https://reqres.in/api/users/2").should((response) => {
expect(response.status).to.eq(204);
});
});
In this example, the cy.request()
function is used to send the request to the endpoint. The response from the API is stored in the response variable and can then be used to write assertions using Chai.js.
Output Of Executed Test Cases
Here is an execution report of test cases from our example:
Join our webinar
Hungry to learn more? Join Ely Lucas and Ambassador Filip Hric as they explore how to easily and efficiently test APIs with Cypress.
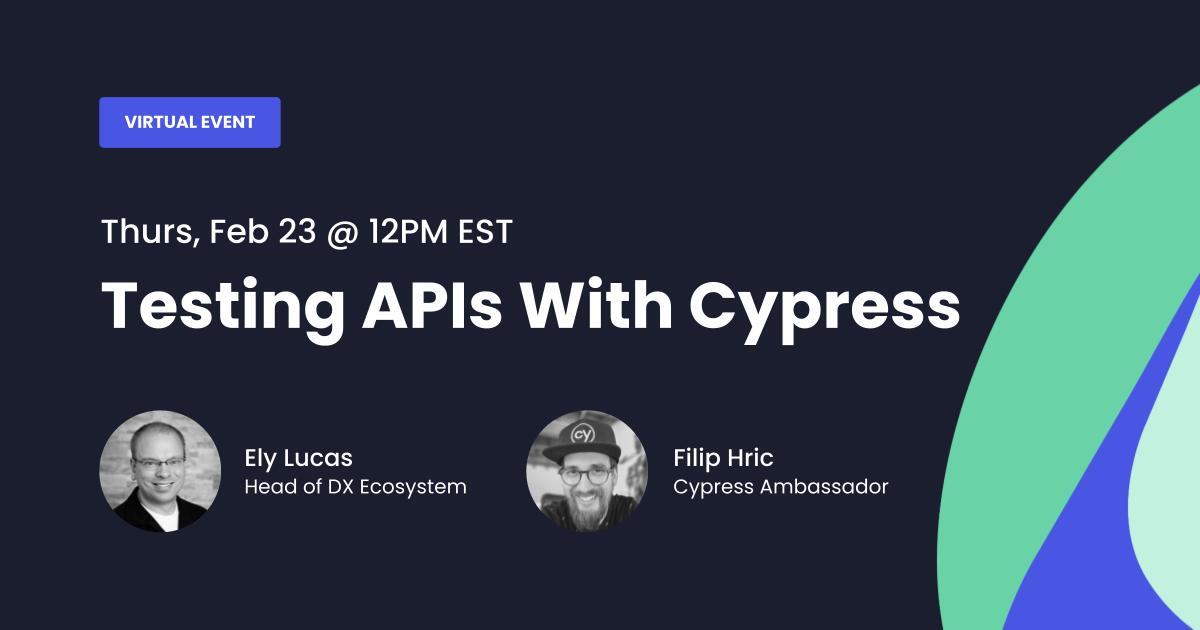
In the next post, we’ll take a look on how to use the Cypress API Plugin improve the testing experience for API tests. Stay tuned!