This is a guest post from Cypress Ambassador Kailash Pathak!
Kailash Pathak is a Sr. QA Lead and 3Pillar Global. Read his blogs at qaautomationlabs.com/blogs. Kailash is 2x AWS | PMI-ACP, ITIL, and PRINCE2 practitioner certified. Check out his blog here.
This post is the second of a series that explores API testing with Cypress. Read the first post on how to automate APIs using Cypress here.
The cypress-plugin-api, written by Cypress Ambassador Filip Hric, is a plugin for effective API testing. Imagine Postman, but in Cypress; this plugin prints out all the information about the API call in the Cypress app UI, providing several benefits:
- In
cypress-plugin-api
, the commandcy.api()
works similarly tocy.request()
. The main difference is that in addition to calling your API,cy.api()
also prints out information about the API call in your Cypress Test Runner. - All of the information can be viewed in time-travel snapshots
- It provides a simple table for viewing cookies, JSON data object and array folding, and color coding of methods in the UI view and in the timeline
The best part: cy.api()
uses cy.request()
in the background. This means you get the same functionality plus a visual UI!
Let's set up the cypress-plugin-api
together
Step 1
Install the plugin using npm or yarn.
npm i cypress-plugin-api
# or
yarn add cypress-plugin-api
Once the API plugin is installed, you can see it in package.json:
Step 2
Next, import the plugin into your cypress/support/e2e.js
file:
import 'cypress-plugin-api'
// or
require('cypress-plugin-api')
Your e2e.js
files will look like this:
Step 3
Create cypress_plugin_api.cy.js
with methods (GET, POST, PUT, DELETE).
For the purposes of this demo, I am taking various API method examples from this example site: https://reqres.in/.
GET Request
it("GET API testing Using Cypress API Plugin", () => {
cy.api("GET", "https://reqres.in/api/users?page=2").should((response) => {
expect(response.status).to.eq(200);
});
});
POST Request
it("POST API testing Using Cypress API Plugin", () => {
cy.api("POST", "https://reqres.in/api/users", {
name: "morpheus",
job: "leader",
}).should((response) => {
expect(response.status).to.eq(201);
});
});
PUT Request
it("PUT API testing Using Cypress API Plugin", () => {
cy.api("PUT", "https://reqres.in/api/users/2", {
name: "morpheus",
job: "zion resident",
}).should((response) => {
expect(response.status).to.eq(200);
});
});
DELETE Request
it("DELETE API testing Using Cypress API Plugin", () => {
cy.api("DELETE", "https://reqres.in/api/users/2").should((response) => {
expect(response.status).to.eq(204);
});
});
API Test Case Execution Report
In the screenshot below, we can see the Body, Response, Headers, and Cookies data displayed in the Cypress App UI. Previously, we had to click on 'inspect' to view this information, but now we have a user interface that allows us to access it easily.
GET Request
POST Request
PUT Request
DELETE Request
Join our webinar
Hungry to learn more? Join Ely Lucas and Ambassador Filip Hric as they explore how to easily and efficiently test APIs with Cypress.
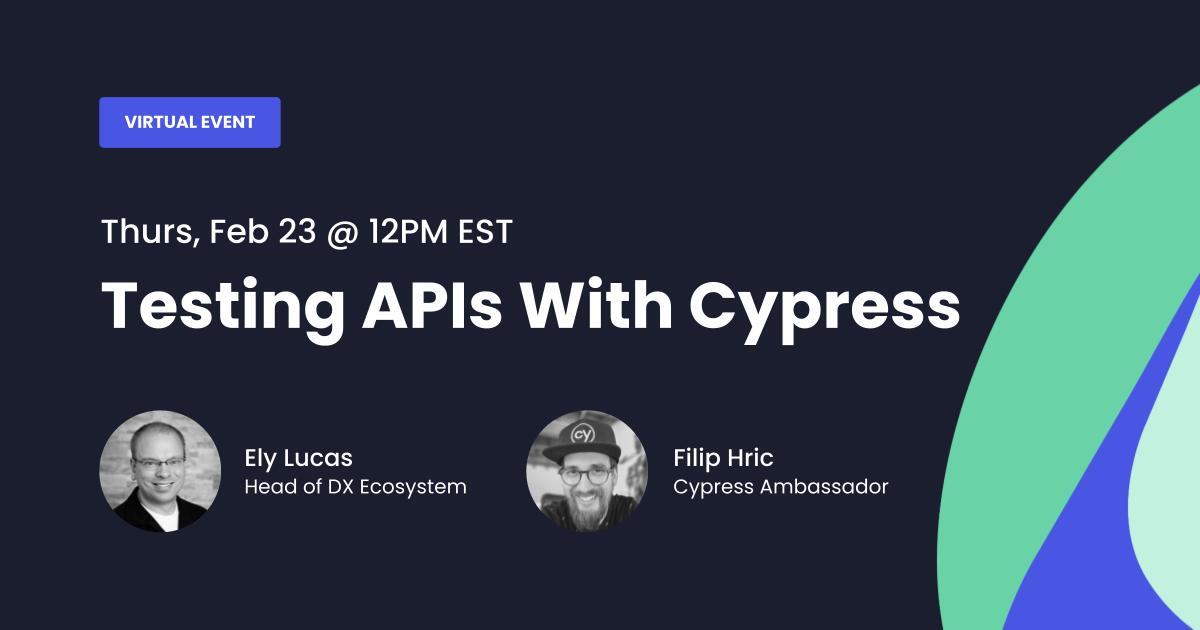
Thanks for tuning in!