Cypress prides itself on providing a first-class experience for Component Testing. Part of this experience is our onboarding process that automatically detects the library and framework used in a project, and scaffolds the relevant files and configuration.
Starting with Cypress 12.7, we are exposing our internal API for the community to leverage and integrate directly into Cypress. This reduces the configuration burden, allowing developers to start testing their components more quickly and easily.
We refer to these APIs as Component Framework Definitions, which are typically <100 lines of code!
Component Integration Testing with Cypress
There are several APIs that Cypress uses to ensure a smooth onboarding experience when configuring and writing component tests.
Typically, once configured, cypress.config
looks something like this for component testing:
import { defineConfig } from 'cypress'
export default defineConfig({
component: {
devServer: {
framework: 'react',
bundler: 'webpack',
},
},
})
And a typical test looks like:
import React from 'react'
import { mount } from 'cypress/react'
import { App } from './App'
it('renders hello world', () => {
mount(<App />)
cy.get('h1').contains('Hello World')
})
When building a Framework Definition, you need to provide the following:
- Requirements: which packages and versions are required?
- Mount Adapter: tell Cypress how to render your library’s components
Framework Definition
The documentation has a detailed description. However, in short, a framework definition can be created with defineComponentFramework
:
export default defineComponentFramework({
type: "cypress-ct-solid-js",
name: "Solid.js",
supportedBundlers: ["vite"],
detectors: [],
dependencies: () => {
return {
type: "solid-js",
name: "Solid",
package: "solid-js",
installer: "solid-js",
description: "A declarative, efficient, and flexible JavaScript library for building user interfaces.",
minVersion: "^1.6.11",
}
}
})
This contains all the information Cypress needs to detect and configure Component Testing with Solid.js.
Mounting Adapter
The final requirement is a Mounting Adapter. A sample one for Solid.js can be found here. It’s only a handful of lines of code:
import { getContainerEl, setupHooks } from "@cypress/mount-utils";
import { render } from "solid-js/web";
let dispose: () => void;
function cleanup() {
dispose?.();
}
interface MountingOptions {
log?: boolean;
}
export function mount(
component: Parameters<typeof render>[0],
options: MountingOptions = {}
) {
// rendering/mounting function.
const root = getContainerEl();
// Render component with your library's relevant
dispose = render(component, root);
return cy.wait(0, { log: false }).then(() => {
if (options.log !== false) {
Cypress.log({
name: "mount",
message: "Mounted component",
});
}
});
}
setupHooks(cleanup);
Demo of Supported Frameworks
Now Solid.js users can enjoy the same polished onboarding experience as our officially supported frameworks:
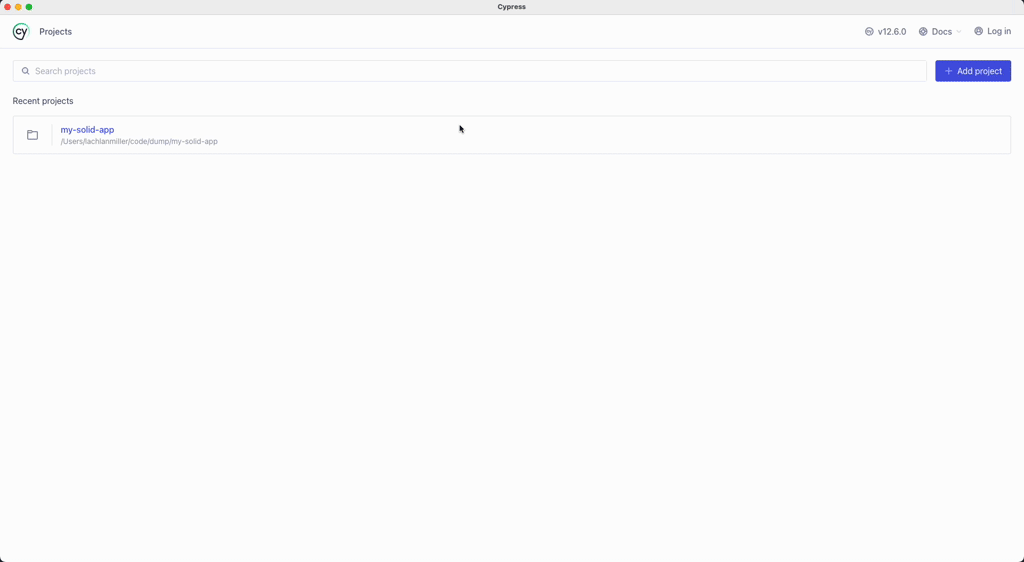
What’s Next?
These APIs are available in Cypress 12.7. There’s still more to come – while developers can easily integrate for libraries and common bundlers, there is still more support required to integrate more complex platforms, such as Nuxt, SvelteKit and Qwik. In a future update to Cypress, the Framework Definition API will be extended for more advanced use cases. Follow our progress on this GitHub issue.
Conclusion
Cypress’s new Component Framework Definition API makes Cypress more extensible as a platform, enabling third parties to provide a more deeply integrated, seamless, and polished experience for component testing and development.
See a full example Framework Definition here, or check the documentation to see how to start writing one for your favorite library and add your own! If you need any help (or just want to say hi!), chat with us in our cypress-frameworks
Discord forum or submit an issue on our Github.
Explore a comprehensive Framework Definition example, or refer to our documentation to learn how to create one for your preferred library and customize it. If you require assistance (or simply want to connect), join our cypress-frameworks Discord forum or submit an issue on our Github repository. Looking to reduce configuration complexities and start component integration testing swiftly? Upgrade to Cypress Cloud today.